Hiding text in images - Steganography
Who would have thought that it was easy to hide text in an image file. At first, I didn’t and then I realized it was exactly what I was looking for in a recent project I was working on. A way to hide information in plain sight.
I remember as a kid, using simple encoding and decoding schemes to share messages with friends. With out a computer, it was a real pain. ‘A’ would be represented by a 1, ‘B’ would be a 2, ‘C’ would be a 3 and so on.
One standard technique is leveraging the Least Significant Bit of the pixels that are in the image. In the end, I needed some simple functions to accomplish this task. The first function would encode the string into an existing image and then the second function would be able to extract the information back out of the file. Now this technique works great for a lossless image types so PNG and BITMAP images are a great place to start. However, using a JPG image is not because since this image type is lossy, meaning the fomat changes pixels based on pixels around it. This feature helps JPG image with compression, but removes a perfect representation of a digital image once transformed into this format.
This approach allows you to easily encode any string that you might have and sort of hide the information.
8 5 12 12 15 0 23 15 18 12 4 = H e l l o W o r l d
Now I thought I could pass messages in grade school and being extremely naive, I assumed no one else would be able to figure it out. How wrong I was when the first person who saw it figured out the ENCODING immediately. Then they were able to DECODE my secret message easily.
Hiding a message in an image is very similar to this approach, it just leverages a few things about colors and how they are stored in a BITMAP. A bitmap has to store pixels and we will start with a small image that is 3 pixels wide and 3 pixels high. All our colors will be represented using hexadecimal notion for a RGB scheme.
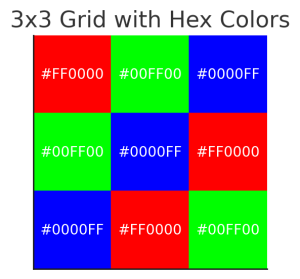
Now the most important thing to notice is that the first two hex numbers represent our red channel, the second two are our green channel and the last two are our blue channel. It is this blue channel that we are going to use for hiding our secret messages, using the least significant bit of a color.
The fact is that a small change in a color is impossible to notice, you just can’t tell the difference. Just for context, the RGB scheme is a 24-bit color model called True Color. It can have 16,777,216 colors which easily covers the entire rainbow. Adding or subtracting 1 from a number value, is impossible for our human eye to notice.
How does this work?
First, you need to be comfortable with binary numbers (at least we won’t be scared of them) to really wrap our hands around how this works. A very simple example will do. In fact. we will be walking through ENCODING only one letter and our 3×3 bitmap above will be a perfect image to use. If you can understand this simple example, it is easy to expand.
The letter M (ASCII 77) in binary = 0 1 0 0 1 1 0 1
Bit 1 | Bit 2 | Bit 3 | Bit 4 | Bit 5 | Bit 6 | Bit 7 | Bit 8 |
---|---|---|---|---|---|---|---|
0 | 1 | 0 | 0 | 1 | 1 | 0 | 1 |
To ENCODE the letter ‘M’ into the image, we are going to use the Least Significant Bit which is the bit farthest to the right and is a part of our blue channel for the pixel color scheme.
Looking at the table below, lets absorb what is really going on for this ENCODING scheme.
PIXEL 1 REVIEW
- The first bit of the letter ‘M’ is a ‘0’.
- Our blue channel in the first row is #00, and the last bit of the blue color is a ‘0’ too.
- Since the letter ‘M’ bit matches the LSB of the blue channel, we don’t even need to adjust the first pixel.
PIXEL 2 REVIEW
- The second bit of the letter ‘M’ is a ‘1’.
- Our blue channel in the first row is #00, and the last bit of the blue color is a ‘0’ too.
- Since the letter ‘M’ bit does not match the LSB of the blue channel, simple change this one bit. We see our pixel color has changed from #00FF00 to #00FF01.
If we continue this pattern for the other 6 bits of the letter ‘M’, we discover that to ENCODE the letter ‘M’ into our image, we didn’t even need to change all of the pixels. Looking at the Translated Blue channel, we can see that we used 8 pixels to represent the letter ‘M’. And no one is any wiser about what we did.
Pixel Index | Hex Color | Blue (Binary) | 8 bits of 'M' | Translated Blue | Final Output Color |
---|---|---|---|---|---|
1 | #FF0000 | 00000000 | 0 | 00000000 | #FF0000 |
2 | #00FF00 | 00000000 | 1 | 00000001 | #00FF01 |
3 | #0000FF | 11111111 | 0 | 11111110 | #0000FE |
4 | #00FF00 | 00000000 | 0 | 00000000 | #00FF00 |
5 | #0000FF | 11111111 | 1 | 11111111 | #0000FF |
6 | #FF0000 | 00000000 | 1 | 00000001 | #FF0001 |
7 | #0000FF | 11111111 | 0 | 11111110 | #0000FE |
8 | #FF0000 | 00000000 | 1 | 00000001 | #FF0001 |
Now this is great, but what happens when we have a long string of characters for ENCODING. Let’s take our ‘Hello World’ for example. It is a total of 11 characters. So to encode this into an image, the image needs to contain at least 88 pixels (11 characters x 8 pixels for each character).
Functions for Delphi
There are plenty of examples for handling this in Python, Javascript, C, C# and other languages and there are even some pretty good videos out there to help.
But I needed this for Delphi and wanted to have the flexibility of working with either BITMAP or PNG files. Since the VCL comes with basic representations of these two image types, it just took a bit of effort to crank this out. Below is everything you need to get this working in Delphi using the standard VCL.
unit Encoding.Image.LSB;
implementation
uses
Vcl.Graphics, // For TBitmap
Vcl.Imaging.pngimage, // For TPNGImage
System.SysUtils, // For common utilities like IntToStr
System.Classes; // For file stream handling (optional)
TRGBTriple = packed record
rgbtBlue: Byte;
rgbtGreen: Byte;
rgbtRed: Byte;
end;
PRGBTripleArray = ^TRGBTripleArray;
TRGBTripleArray = array[0..MaxInt div SizeOf(TRGBTriple) - 1] of TRGBTriple;
procedure HideDataInPNG(const ImageFile, OutputFile: string; const Data: string);
procedure HideDataInBitmap(Bitmap: TBitmap; const Data: string);
function ExtractDataFromBitmap(Bitmap: TBitmap; DataLength: Integer): string;
function ExtractDataFromPNG(const ImageFile: string; DataLength: Integer): string;
interface
procedure HideDataInPNG(const ImageFile, OutputFile: string; const Data: string);
var
Bitmap: TBitmap;
PNG: TPNGImage;
begin
Bitmap := TBitmap.Create;
PNG := TPNGImage.Create;
try
// Load the PNG file into a TBitmap
PNG.LoadFromFile(ImageFile);
Bitmap.Assign(PNG);
// Use the existing HideDataInBitmap logic
HideDataInBitmap(Bitmap, Data);
// Save the modified bitmap back to a PNG file
PNG.Assign(Bitmap);
PNG.SaveToFile(OutputFile);
finally
Bitmap.Free;
PNG.Free;
end;
end;
procedure HideDataInBitmap(Bitmap: TBitmap; const Data: string);
var
x, y, DataIndex, BitIndex: Integer;
Row: PRGBTripleArray;
CharValue: Byte;
DataToHide: string;
begin
Bitmap.PixelFormat := pf24bit; // Ensure 24-bit format for direct pixel access
// Append a null terminator to the data
DataToHide := Data + #0;
DataIndex := 1; // Start with the first character
BitIndex := 1; // Start with the first bit of the character
for y := 0 to Bitmap.Height - 1 do
begin
Row := Bitmap.ScanLine[y]; // Get a pointer to the row of pixels
for x := 0 to Bitmap.Width - 1 do
begin
if DataIndex > Length(DataToHide) then Break;
CharValue := Byte(DataToHide[DataIndex]);
// Embed the current bit in the LSB of the blue channel
Row[x].rgbtBlue := (Row[x].rgbtBlue and $FE) or ((CharValue shr (8 - BitIndex)) and $01);
Inc(BitIndex); // Move to the next bit
if BitIndex > 8 then
begin
BitIndex := 1; // Reset for the next character
Inc(DataIndex); // Move to the next character
end;
end;
if DataIndex > Length(DataToHide) then Break;
end;
end;
function ExtractDataFromPNG(const ImageFile: string; DataLength: Integer): string;
var
Bitmap: TBitmap;
PNG: TPNGImage;
begin
Bitmap := TBitmap.Create;
PNG := TPNGImage.Create;
try
// Load the PNG file into a TBitmap
PNG.LoadFromFile(ImageFile);
Bitmap.Assign(PNG);
// Use the existing ExtractDataFromBitmap logic
Result := ExtractDataFromBitmap(Bitmap, DataLength);
finally
Bitmap.Free;
PNG.Free;
end;
end;
function ExtractDataFromBitmap(Bitmap: TBitmap; DataLength: Integer): string;
var
x, y, DataIndex, BitIndex: Integer;
Row: PRGBTripleArray;
ExtractedChar: Byte;
ExtractedData: string;
IsNullFound: Boolean;
begin
Bitmap.PixelFormat := pf24bit; // Ensure 24-bit format for direct pixel access
DataIndex := 1; // Start with the first character
BitIndex := 1; // Start with the first bit of the character
ExtractedChar := 0; // Initialize to hold the reconstructed character
ExtractedData := ''; // Initialize the result string
IsNullFound := False;
for y := 0 to Bitmap.Height - 1 do
begin
Row := Bitmap.ScanLine[y]; // Get a pointer to the row of pixels
for x := 0 to Bitmap.Width - 1 do
begin
// If length is provided, stop after extracting the specified number of characters
if (DataLength > 0) and (DataIndex > DataLength) then
Break;
// Extract the LSB of the blue channel
ExtractedChar := ExtractedChar or ((Row[x].rgbtBlue and $01) shl (8 - BitIndex));
Inc(BitIndex); // Move to the next bit
if BitIndex > 8 then
begin
// Complete the character and append to the result
if ExtractedChar <> 0 then
ExtractedData := ExtractedData + Char(ExtractedChar);
// Reset for the next character
if ExtractedChar = 0 then IsNullFound := True;
// Reset for the next character
ExtractedChar := 0;
BitIndex := 1;
Inc(DataIndex); // Move to the next character
if IsNullFound then Break;
if (DataLength > 0) and (DataIndex > DataLength) then Break;
end;
end;
if IsNullFound then Break;
if (DataLength > 0) and (DataIndex > DataLength) then Break;
end;
Result := ExtractedData; // Return the extracted data
end;
end.